JavaScript arrays are versatile data structures that serve as the backbone for managing data in simple scripts and complex web applications.
console.log()
It is a simple yet powerful tool for efficiently debugging arrays and understanding their structure.
This article’ll explore how to log arrays effectively using console.log()
its advanced features.
Why use console.log()
for Arrays?
The console.log()
method allows us to output JavaScript values to the console for inspection. When working with arrays, it becomes necessary for tasks such as:
- Inspecting the structure of arrays.
- Debugging array manipulation logic.
- Tracking changes during transformations (e.g., using
map
,filter
, orreduce
).
Examples of Logging Arrays in the Console
Log the Entire Array
The simplest way to inspect an array is by logging it directly:
const blogSites = ["HoverConsole", "MDN", "W3Schools", "GeeksforGeeks"];
console.log("Popular blog and documentation sites:", blogSites);
This method is ideal when you want a quick look at the entire array structure.
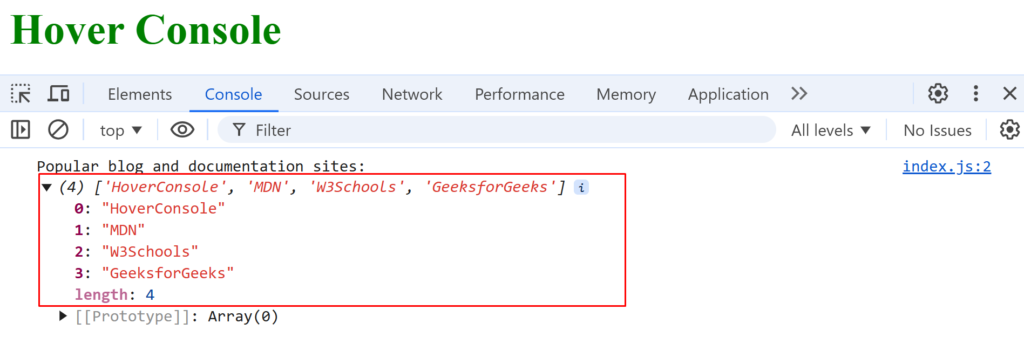
Log Specific Elements
Sometimes, you only need to inspect specific elements. Accessing elements by their indices is straightforward:
const colors = ["Red", "Green", "Blue", "HoverConsole"];
console.log(colors[3])
This approach is particularly useful when debugging array operations or verifying specific data points.
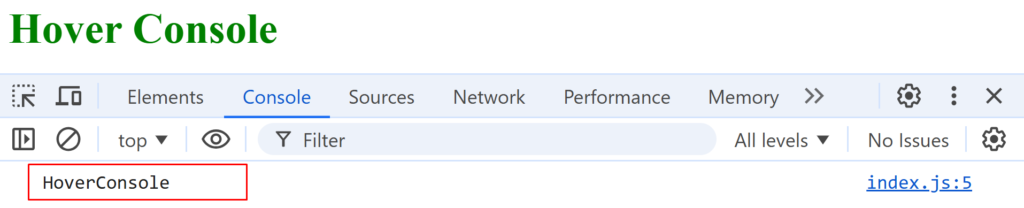
Log Each Element Using a Loop
To log every element individually, you can use loops like forEach
:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(num => console.log(num));
This method is concise and efficient, making it a popular choice for debugging arrays.

For more control, a traditional for
loop works well:
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(`Element ${i}:`, numbers[i]);
}
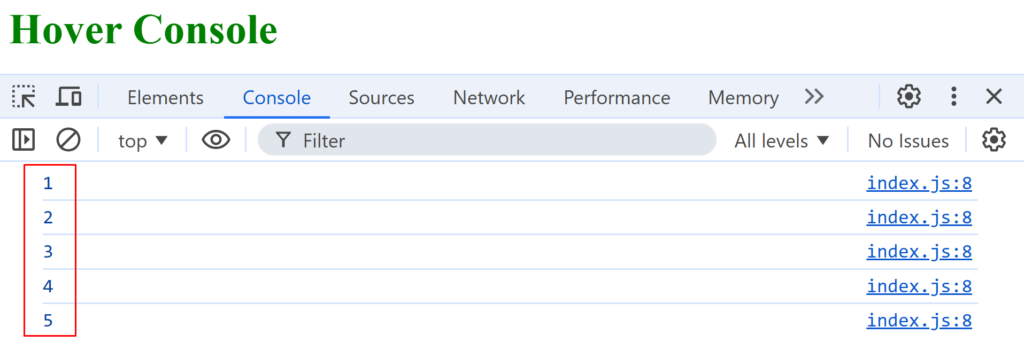
Log Nested Arrays
Nested arrays, or multidimensional arrays, can be logged directly to understand their structure:
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
];
console.log(matrix);
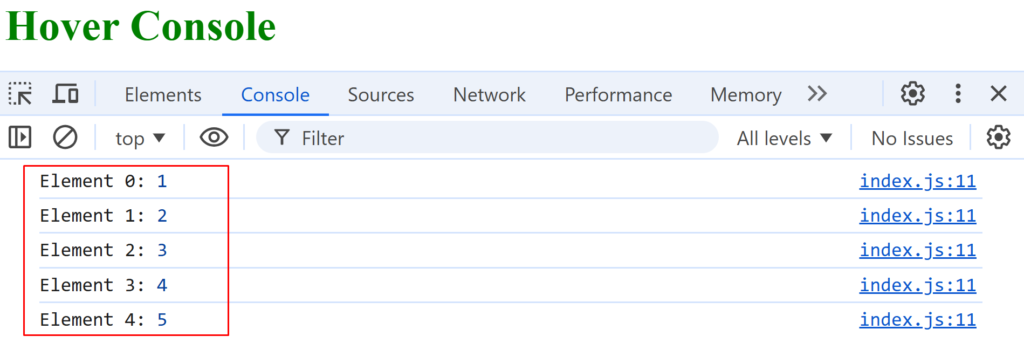
To inspect individual rows or elements, use nested loops:
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
];
matrix.forEach((row, rowIndex) => {
console.log(`Row ${rowIndex}:`, row);
});
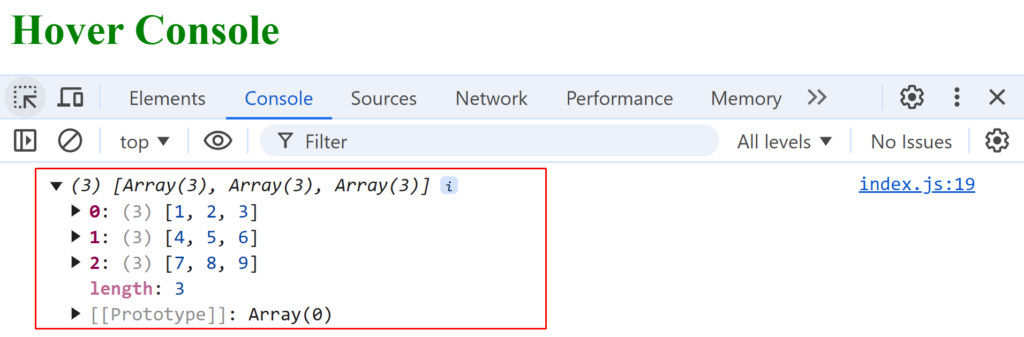
Log Array of Objects with console.table()
For arrays of objects, console.table()
provides a clean, tabular format:
const users = [
{ name: "Alice", age: 25, site: "hoverConsole" },
{ name: "Bob", age: 30, site: "MDN" },
];
console.table(users);
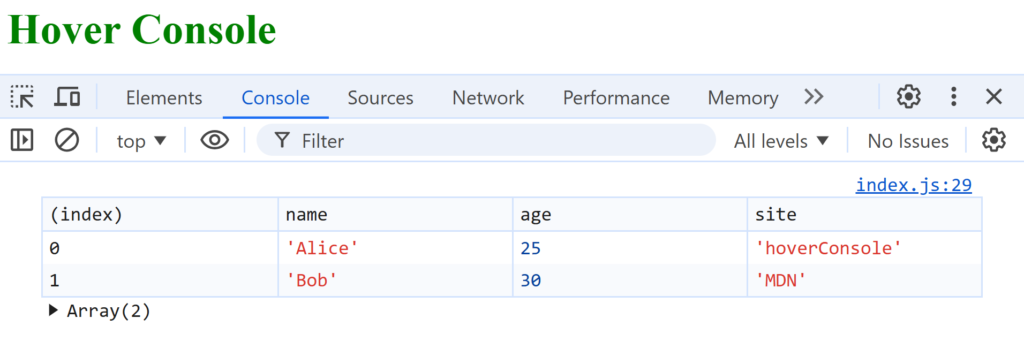
This approach is especially helpful when debugging API responses or datasets.
Styled Log for Arrays
To make logs more visually distinct, use %c
for styling:
const resources = ["MDN", "W3Schools", "hoverConsole"];
console.log("%cLearning Resources:", "color: blue; font-weight: bold;", resources);
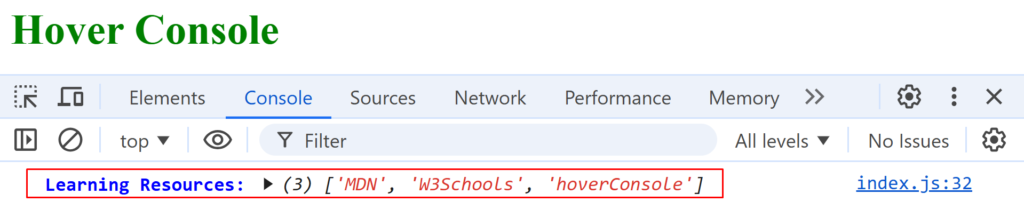
This is a great way to highlight specific logs during a debugging session.
Advanced Tips for Logging Arrays
Logging Array Transformations
When applying methods like map()
, filter()
, or reduce()
, logging intermediate results can clarify your understanding:
const numbers = [1, 2, 3];
const squared = numbers.map(num => num ** 2);
console.log("Original array:", numbers);
console.log("Squared array:", squared);
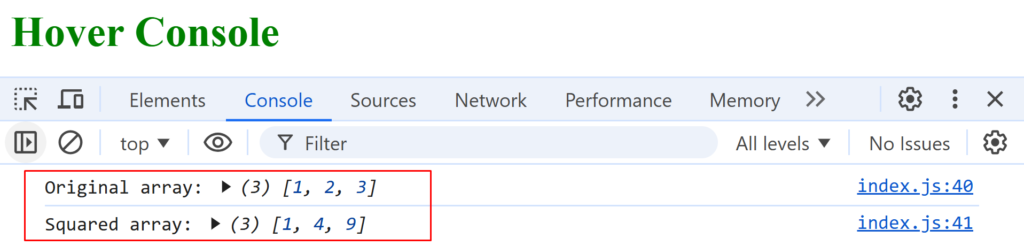
Debugging Undefined or Null Values
Undefined or null elements in arrays can lead to unexpected issues. Logging the array helps you catch these:
const data = [1, undefined, 3, null];
console.log(data);
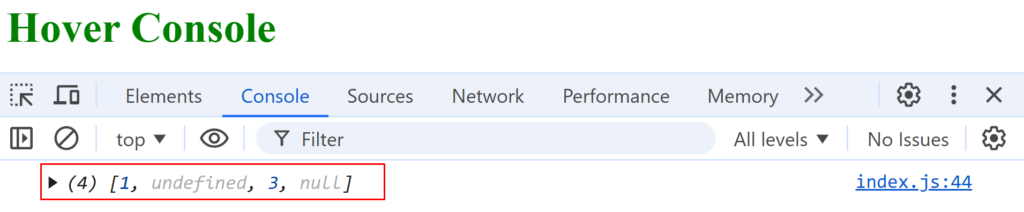
Grouping Logs
For cleaner debugging, group related logs together:
const tools = ["hoverConsole", "debugger", "console.table"];
const descriptions = tools.map(tool => `${tool} is a powerful debugging tool`);
console.group("Debugging Tools Inspection");
console.log("Original tools array:", tools);
console.log("Descriptions array:", descriptions);
console.groupEnd();
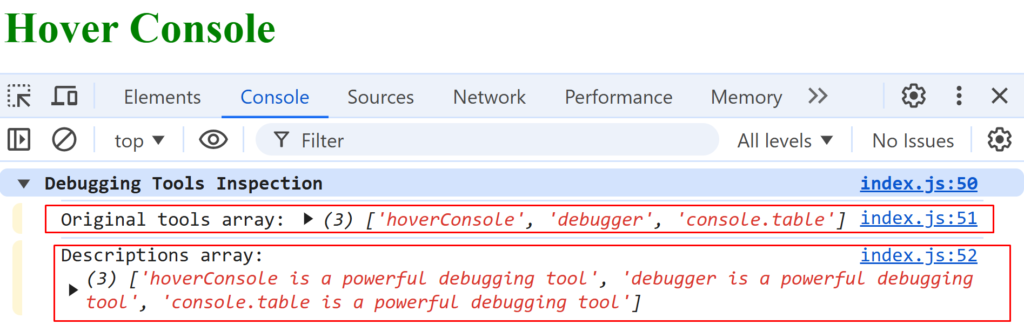
Logging Large Arrays
When dealing with large arrays, logging only a slice can prevent console clutter:
const largeArray = Array.from({ length: 1000 }, (_, i) => `hoverConsole-${i + 1}`);
console.log("First 10 elements:", largeArray.slice(0, 10));
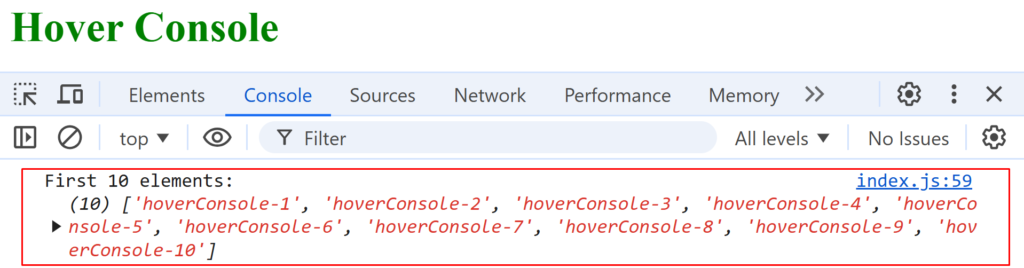
Best Practices for Using console.log()
with Arrays
- Add Descriptive Context: Always accompany your logs with meaningful messages for clarity.
- Use
console.table()
When Appropriate: It’s often more readable for an array of objects. - Avoid Excessive Logging: Too many logs can clutter your console and make debugging harder.
- Clear Logs When Necessary: Use
console.clear()
at the start of a debugging session to avoid confusion. - Explore Alternatives: For complex debugging, tools like breakpoints can compliment
console.log()
.
Conclusion
console.log()
is a powerful debugging tool for JavaScript arrays, enabling efficient inspection of simple arrays, nested structures, and transformations to enhance code understanding and debugging.
Happy coding!
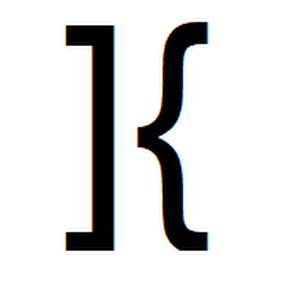
He is the founder of Hover Console.
Khalid began his career as a software engineer in 2003. He leads strategic initiatives, guiding Hover Console from start to finish, driving progress in software development. Passionate about using technology for positive change, Khalid excels in creating innovative solutions. He’s committed to collaboration, diversity, industry advancement, and mentoring aspiring developers.