Two widely used methods from the console object are console.log()
and console.debug()
. Though similar, subtle differences can impact when and how each should be used.
Understanding these distinctions improves the debugging process by ensuring that developers can log the appropriate level of detail during development, which in turn helps maintain clean and efficient code.
What is console.log()?
The console.log()
function is widely used in web development to display messages, object data, and variable values in the browser’s console, helping developers debug and track execution.
In JavaScript development, debugging is essential for ensuring code behaves as expected. Developers usually use console.log()
for:
Displaying general message.
//Displaying a general message
console.log("Welcome to Hover Console");

Tracking application states
// Tracking blog post status
let postStatus = "Writing a Post for the 'Hover Console' Blog";
console.log("Current Post Status:", postStatus);
// Simulating a status update
postStatus = "Ready to publish on 'Hover Console' Blog";
console.log("Post Status Updated:", postStatus);

Debugging errors and warnings.
// Debug warnings and errors related to posts
let postCount = 0;
if (postCount === 0) {
console.log("No Posts Yet! Start Writing Your First Blog Post on 'Hover Console Blog'.");
}
try {
// Simulating an error in blog post publishing
let result = undefinedFunction(); // This will throw an error
} catch (error) {
console.log(
"An Error Occurred While Publishing the Blog Post on 'Hover Console' Blog",
error.message
);
}

Showing the Dynamic Values of the Variables
// Showing dynamic values of blog post details
let blogPost = {
id: 1,
title: "Why You Should Use the 'Hover Console Website' in Your Daily Life",
author: "Alice",
status: "Published",
wordCount: 1200,
};
console.log("Blog Post Details:", blogPost);
console.log(
`The blog post titled "${blogPost.title}" by
${blogPost.author} contains ${blogPost.wordCount} words.`
);
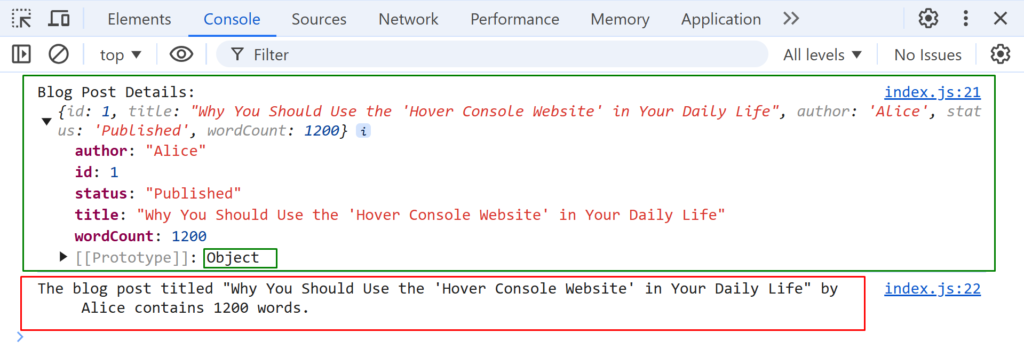
Actually, console.log()
is used for debugging because it is simple and easy to use. It prints all messages without any browser’s logging level settings.
What is console.debug()?
console.debug()
is designed for detailed, low-level debugging, similar to console.log()
. It allows developers to log messages but is typically reserved for less critical outputs.
This is useful for keeping the console focused on more critical information while allowing detailed debugging when needed. console.debug()
is generally used to output less critical messages and is intended for more in-depth debugging scenarios.
console.debug("Hello there, Welcome to Hover Console - ready to assist with deubug");
The key difference is that console.debug()
depending on browser settings, it is less visually prominent and may be hidden by default, making it ideal for non-critical, detailed debugging messages.
Many browsers allow logging level adjustments (e.g., Errors, Warnings, Info, Verbose), and console.debug()
messages appear mainly when set to “Verbose” or “Debug,” helping developers focus on key issues.

Key Differences Between console.log() and console.debug()
Visibility in the Console
One of the main differences between console.log()
and console.debug()
is their visibility in the console. Console.log ()
output messages to the console that are always visible unless specifically filtered by the developer. These messages can appear at any logging level setting.
On the other hand, console.debug()
is often showing a message by default in certain browser environments unless the logging level is set to “Verbose” or “Debug”. This makes console.debug()
less interfering, which is particularly useful for situations where developers want to log more detailed information
Purpose and Use Cases
console.log()
is ideal for general logging, helping developers track application flow, inspect variables, or log key information. It ensures important details are visible to anyone reviewing the console, regardless of logging settings.
Example use case: Logging key events in an application, such as user interactions, application states, or API call responses.
function handleUpdateButton() {
console.log("Welcome to the Hover Console Website!");
}
console.debug()
is best used for low-level, in-depth debugging. This method is designed for scenarios where detailed information is required but doesn’t need to be visible in all logging situations. It allows developers to focus on specific aspects of the code without cluttering the console.
For example, developers might use console.debug()
when troubleshooting complex logic or testing particular code paths, ensuring the console isn’t overwhelmed with excessive information.
This feature makes it especially useful during intensive debugging sessions where detailed insights are necessary.
function fetchDemoData() {
let data = apiCallDemo();
console.debug("Data fetched:", data);
}
Behavior Across Browsers
Another important distinction is how these methods behave across different browsers. In most modern browsers, console.log()
behaves consistently—messages are always logged to the console unless explicitly filtered out. However, console.debug()
may behave differently depending on the browser’s settings.
In Chrome and Firefox, console.debug()
logs are often hidden unless the console is set to “Verbose” or “Debug.” It may behave like this in other browsers due to the lack of such settings. Developers should consider these inconsistencies when choosing between the two methods.
Visual Prominence
As previously mentioned, console.debug()
logs are less prominent and often appear in lighter colors, like in Chrome.
This subtle visual difference helps distinguish messages, aiding in managing console output during complex debugging.
When to Use console.log() vs. console.debug()
The choice between console.log()
and console.debug()
ultimately depends on the specific needs of the project and personal or team preferences. Here are a few guiding principles to help make the decision easier.
Here’s a table comparing the usage of console.log()
and console.debug()
:
Criteria | console.log() | console.debug() |
---|---|---|
Purpose | General-purpose logging of important messages and key events | Detailed debugging of specific sections of code |
Visibility | Visible by default in most browser developer consoles | May be hidden in certain browsers unless explicitly enabled |
Use Case | Tracking important application state changes, user interactions, etc. | want to know extra details for debugging |
Prominence | Logs messages prominently for all developers and testers | Log messages with lower prominence. |
Criticality | Used for critical application flow data | Used for critical application flow and more detailed information |
Summary
Both console.log()
and console.debug()
are valuable tools for logging and debugging in web development, but they serve distinct purposes.console.log()
is a general-purpose, widely used method suitable for scenarios where visible and easily accessible logs are needed.
On the other hand, console.debug()
is best used for minimal, comprehensive debugging. Because of its less noticeable display, developers may concentrate on important details without imposing the output, keeping the console cleaner.
By understanding these differences and using each method appropriately, developers can optimize their debugging process, ensuring cleaner, more organized code and efficient problem-solving.
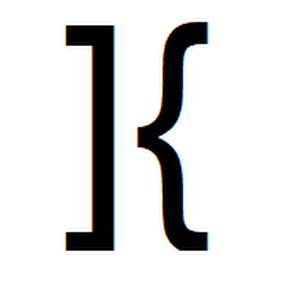
He is the founder of Hover Console.
Khalid began his career as a software engineer in 2003. He leads strategic initiatives, guiding Hover Console from start to finish, driving progress in software development. Passionate about using technology for positive change, Khalid excels in creating innovative solutions. He’s committed to collaboration, diversity, industry advancement, and mentoring aspiring developers.