JavaScript provides developers with a variety of console methods for debugging and logging. Among these, console.info
and console.log
are widely used. Understanding their subtle differences can enhance debugging and improve code clarity.
What is console.log
?
console.log
is the most widely used method in JavaScript for printing messages to the console. It is versatile and can display strings, objects, arrays, and other data types.
Common Use Cases
- Debugging code by logging variable values.
- Tracking the flow of execution.
- Printing messages for developers during development.
Example Usage
let learningSiteInfo = { name: "Hover Console", type: "website" };
console.log("Learning Site Details:", learningSiteInfo);
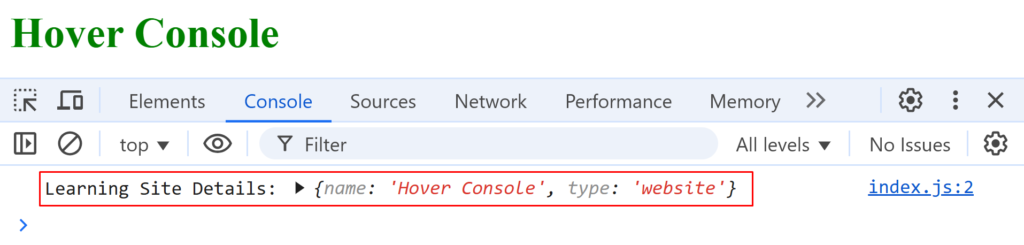
What is console.info
?
Definition and Purpose
console.info
is a method specifically designed for informational messages. It functions similarly to console.log
but is intended to convey non-critical information.
Common Use Cases
- Highlighting informational messages.
- Providing context or metadata about operations.
Example Usage
console.info("Retrieving user data from the Hover Console database...");
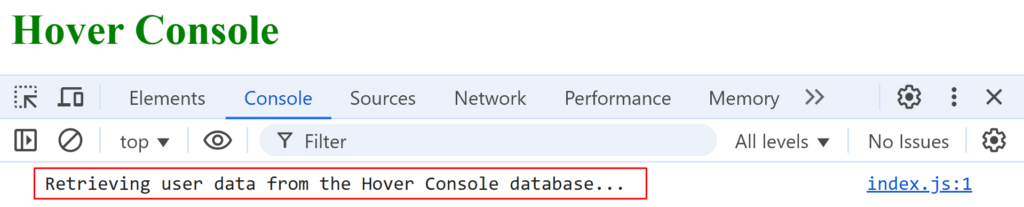
Key Differences Between console.info
and console.log
Functional Differences
Both methods log messages to the console, but console.info
is semantically intended for informational purposes, while console.log
is more generic.
Visual Differences in Browsers/Environments
In some browsers, console.info
messages are styled differently. However, in environments like Node.js, they may appear identical.
Differences in Log Levels
console.log
are often categorized under “debug” or “log” levels.console.info
is categorized under the “info” level in structured logging systems.
When to Use console.info
vs console.log
Choosing Based on Context
- Use
console.log
for general debugging and variable inspection. - Use
console.info
to convey important but non-critical information.
Best Practices for Effective Debugging
- Maintain consistency by using
console.info
informational logs. - Avoid overloading the console with unnecessary logs.
Browser and Environment Support
How console.info
and console.log
are Handled Across Browsers
Most modern browsers support both methods. However, the visual distinction between them may vary.
Behavior in Node.js
In Node.js, console.info
and console.log
typically behave the same way, with no visual differentiation.
Performance Considerations
Impact on Application Performance
Excessive use of console.log
or console.info
can slow down your application, especially in production environments.
Minimizing Console Usage in Production
- Use environment-based logging to disable console methods in production.
- Rely on structured logging libraries for production-grade logging.
Common Misuses
Misinterpretation of console.info
as a Replacement for console.log
Some developers use console.info
interchangeably with console.log
, missing its semantic purpose.
Overusing Both Methods in Production Code
Leaving excessive logs in production can clutter the console and expose sensitive information.
Practical Examples
Debugging a Web Application
console.log("User clicked the 'Publish' button on the Hover Console blog.");
console.info("Clicked Button ID: btn-publish");
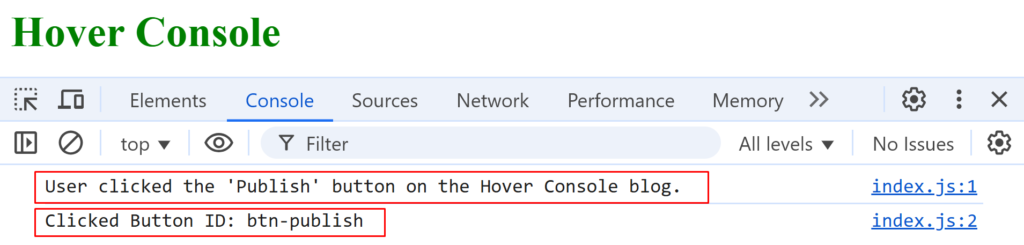
Structured Logging with console.info
console.info("[INFO] Data fetch started at", new Date().toISOString());
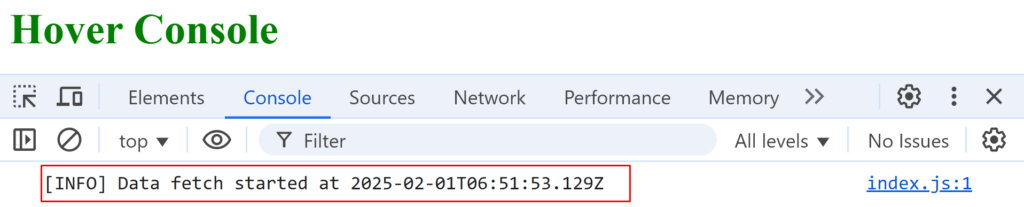
Comparing Outputs in a Real Scenario
const user = {
id: 1,
name: "John Doe",
email: "john.doe@example.com",
role: "Author",
bio: "Tech enthusiast and blogger at Hover Console.",
joinedAt: "2024-01-15",
posts: [
{
id: 101,
title:
"JavaScript Debugging: 5 Essential Techniques for Effective Error Resolution",
published: true,
},
{
id: 102,
title:
"console.log() vs console.debug(): for Efficient JavaScript Debugging",
published: false,
},
],
};
console.log("Hover Console Blogsite: Debugging user data:", user);
console.info("Hover Console Blogsite: User data loaded successfully.");
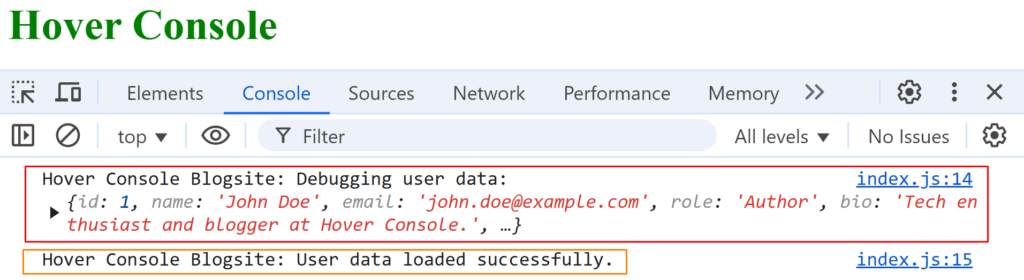
Advanced Debugging Tools
Modern IDEs and browser developer tools offer breakpoints, watch expressions, and performance profiling, reducing reliance on console methods.
Here’s a concise table summarizing the differences between console.info
and console.log
:
Aspect | console.log | console.info |
---|---|---|
Purpose | General-purpose logging for debugging and variable inspection. | Logging informational messages or metadata. |
Semantic Meaning | No specific semantic meaning, used for all types of logs. | Intended for non-critical informational messages. |
Log Level | Categorized under “debug” or “log” levels. | Categorized under “info” level in structured logging. |
Common Use Cases | Debugging variables, tracking execution flow, printing outputs. | Highlighting important information, status updates. |
Behavior in Node.js | Same as console.info (no visual distinction). | Same as console.log (no visual distinction). |
Performance Impact | Similar to console.info ; excessive use can slow down performance. | Similar to console.log ; avoid overuse in production. |
Best Practice | Use for general debugging purposes. | Use to log significant but non-critical messages. |
Understanding the differences between console.info
and console.log
helps developers write clearer, more maintainable code. While both methods log messages to the console, their semantic purposes differ.
Use console.log
for general debugging and console.info
for conveying informational messages. Adopting best practices and leveraging advanced tools can further enhance your debugging process.
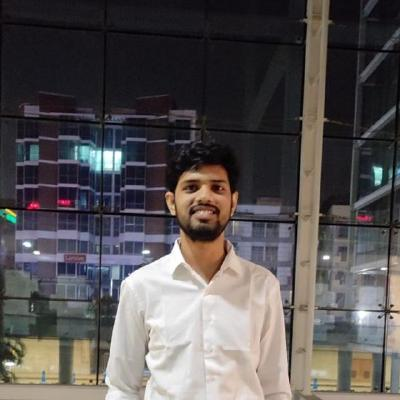
Frontend Software Engineer skilled in JavaScript and TypeScript, with expertise in crafting responsive web interfaces. Also a Content Writer, combining technical knowledge with storytelling to deliver engaging, informative articles for developers and tech enthusiasts.