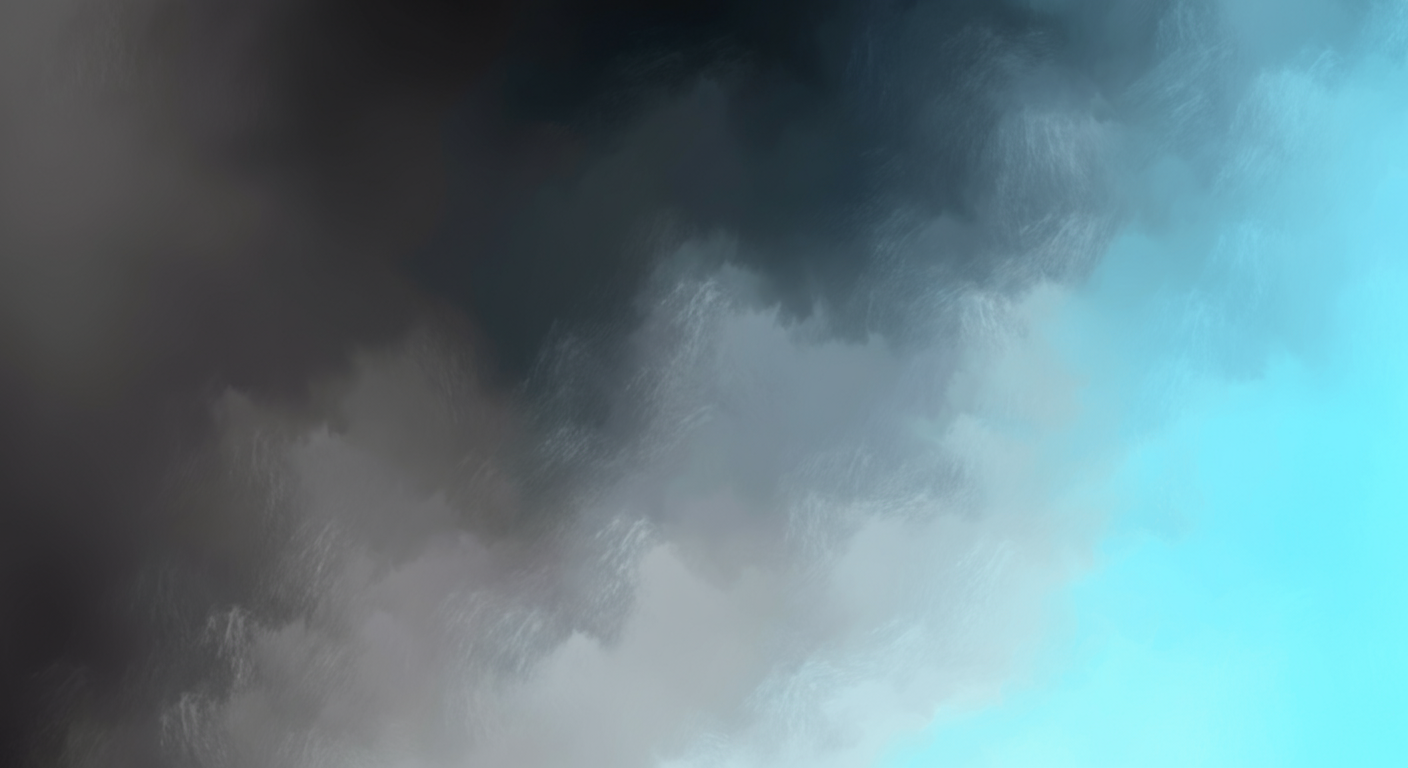
CARI KAMI DI GOOGLE "OLE777"
〰️
CARI KAMI DI GOOGLE "OLE777" 〰️
Mainkan Game Slot Demo Spaceman X500 Pragmatic Terbaru 2025
Selamat datang di situs slot demo spaceman x500 terbaru di indonesia dengan jutaan bonus setiap harinya. Bermain game demo slot spaceman pragmatic play merupakan pilihan terbaik jika anda ingin maxwin dengan mudah. Dengan bonus slot demo x500 anda akan mendapatkan kemenangan dengan mudah, oleh sebab itu join sekarang.